Becoming a Full Stack Developer in 2024
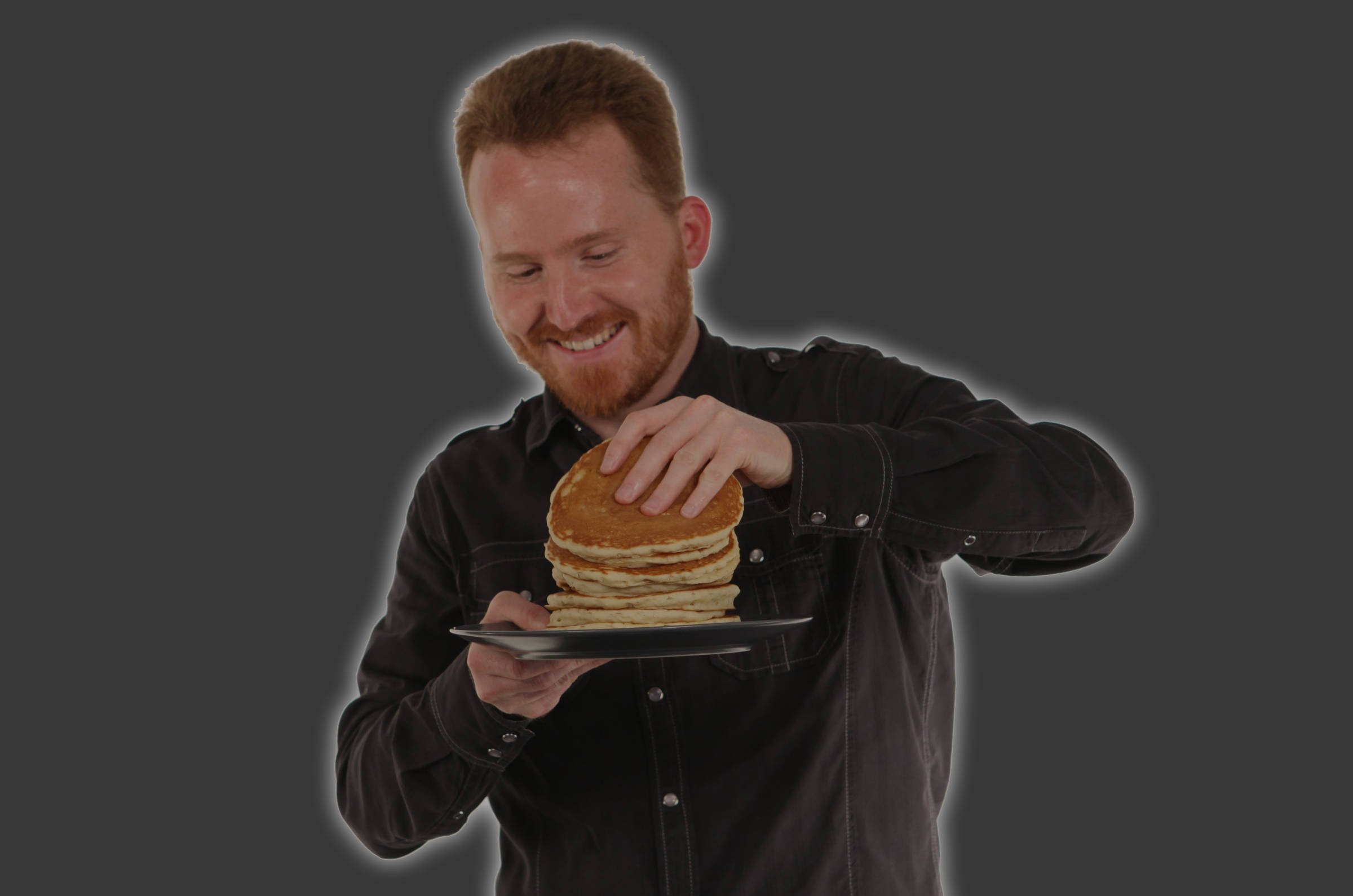
Table of Contents
Intro
How to Learn
Learning the Fundamentals
Basics of Vanilla Frontend
Choose a Frontend Framework
SQL Database Development
ASP.NET MVC Backend
A CMS as a Backend
Node or Serverless Backend
DevOps
Suggested Next Steps
Recently, somebody asked how I’d recommend becoming a full stack developer if I were to start over in 2024, and this article is an attempt to answer that question. Being a full stack developer can mean a lot of things, so I’ll define it according to my experiences:
“A full stack developer is a person capable of working in all the technologies needed to build a website, including the database, the backend, the frontend, and the deployment process.”
That’s a pretty generic definition, so I’ll focus on this article on the stacks I’m more familiar with:
“A full stack developer is familiar with SQL databases, ASP.NET MVC and C# on the backend, vanilla frontend (HTML, CSS, JavaScript), and framework-based frontends (React, Angular, Svelte). On top of that, content management systems (e.g., Umbraco, Contentful, Kentico), and an automated deployment platform (e.g., TeamCity, Azure DevOps, GitHub Actions, Bitbucket Pipelines, and so on).”
This is by no means the only stack, but you will find that many of the other stacks out there have much in common with this one.
How to Learn
Before diving into specifics, it’s worth considering how you’ll want to learn, as becoming a full stack developer can require a considerable investment of your time. It’s also important to set expectations, as you won’t become a master full stack developer right away; it will take a career to refine your skills. That said, don’t let that discourage you, as you can certainly become a valuable contributor in just a few months!
The path you take also depends on your experiences so far. For example, if you have already graduated with a degree in computer science, that is a huge leg up in terms of understanding the fundamentals. On the other hand, much of what is learned in college is not directly related to becoming a full stack developer, and so it isn’t necessary to invest several years of your life into something that you can otherwise achieve in much less time. Or, as Matt Damon would say:
“You wasted $150,000 on an education you coulda got for $1.50 in late fees at the public library.”
This very much depends on your end goals and how much of a self-directed learner you are. There are also bootcamps that teach you many of the basics in a more structured environment without going so far as the full college experience.
To put this another way, I spent very little time doing any actual web development in college. I did do some Java in some classes, but I have never used Java in a professional context. There were also some classes that made use of HTML, JavaScript, and CSS, but those technologies were not the focus of those classes. Excluding classes that were not directly related to web development (e.g., history, math, volleyball, ethics, video art, speech, etc.), even the ones somewhat closely related to web development weren’t of immediate practical use (e.g., compiler construction).
If you are a self-directed learner and don’t mind a lot of reading, you can certainly read a few books and get most of what you need. There are also online tutorials (e.g., YouTube, LinkedIn Learning, Egghead.io, Udemy, and so on) to help supplement your learning.
No matter the path that you take, I do recommend practically applying your skills as you learn them, as this will help your retain the knowledge and to be more effective as a full stack developer. There are many ways of doing this, with one of the more common approaches being to build your own portfolio website using the technologies you are learning.
Another approach you can take is to build a few projects as you learn, perhaps even as open source. Taking this a step further, you might also write some articles as you go along to share what you have learned. Not only does this help others on a similar journey, but it can show off what you are learning and help to cement the knowledge in your own mind, not to mention serve as a reference for your future self.
Learning the Fundamentals
When becoming a full stack developer, there are the obvious things you’ll want to learn, such as backend and frontend. However, what about the underlying concepts that help you when working on the day-to-day tasks that go beyond just the syntaxes of the programming languages you are working with? These are what are called fundamentals, and they are a great help to any programmer when writing code.
College can be a great place to learn these fundamentals, but I’ll go out on a limb and guess you don’t want to spend four years and a hundred grand on them. Assuming that’s the case, here’s another approach.
Learn the fundamentals by first finding out what the fundamentals are, and then by researching the ways that you want to learn them. I’ll give you a list here of things I consider fundamental as a starting point for your research:
Data Structures & Algorithms. These two are usually mentioned at the same time, as both require the other to be of any use. After all, what use is a binary search if you don’t have a binary tree to search (aside: you can actually use a binary search on things other than binary trees, but those too would be data structures)? Some examples of data structures: arrays, lists, sets, dictionaries, linked lists, stacks, trees, graphs, tuples, and even more abstract structures like enumerables. Some examples of algorithms, at a high level, along with concrete samples: searching (first that matches a condition), sorting (ascending), replicating (an exact copy), filtering (all products under a max price), matching (intersection of two sets), and calculating (digits of pi). Eventually, concepts like recursion will become second nature, and you’ll know when a more iterative approach is preferred.
Complexity. Learn how to analyze the complexity and resource usage of your code. Big-O notation is a popular approach for time complexity.
Design Patterns. These are common ways of approaching a given task. One of the most common design patterns is the Singleton pattern, which allows you to create a single instance of a type without allowing others to be created (that’s how you end up with the clone wars, and nobody wants that).
Code Compilation. This means the process of converting the text that you type into the machine code that instructs computers what to do. This has its own value in terms of informing the algorithms you write, but is especially relevant to web development. One example would be that you can pass a string to a function in JavaScript, and that string can be parsed into HTML elements. The terms you will want to know about include: compilation, parsing, transpiling, and interpreted languages.
Computer Hardware. Knowing how hardware works greatly impacts the algorithms and data structures you write. For example, the speed profiles of registers, RAM, and drives impact where you allocate memory. And the differences between code that is run on a CPU vs code that is run on a GPU can impact your programming decisions.
Networking. This includes the physical side of things (modems, routers, clients/servers), the infrastructure elements (DNS, ISPs, latency, throughput), and the protocols (TCP/IP, HTTP/2, HTTPS, gzip/brotli compression). You’ll probably also want to know about more modern aspects that are now commonplace, such as proxy CDNs.
Object-oriented programming (OOP). Regardless of the programming language, OOP includes fairly universal concepts such as encapsulation, abstraction, inheritance, and polymorphism.
This list might seem intimidating, but it’s also worth mentioning you wouldn’t typically try to learn all of this before learning other aspects of web development. More likely, you’ll start with something more concrete and practical (e.g., displaying a webpage), and then revisit some of these more involved fundamentals.
This will look different for everyone, but you could start with a book on learning HTML, CSS, and JavaScript, then later read a book on design patterns. Then off to a book on React, and then back to a book on data structures & algorithms. Then a book on ASP.NET MVC, followed up by a book on the history of computing. And so on.
In other words, this will not be a linear journey. You will bounce back and forth between concepts, probably not fully understanding them as you go. As you learn more things, other things you previously learned will gain new meaning. If you create a checklist of the above concepts and can check off all of them (or even most of them), you will have become an invaluable developer, no matter the programming languages you end up using.
Basics of Vanilla Frontend
There are a million options to choose from when it comes to frontend frameworks. These include things like React, Angular, Svelte, Boostrap, Tailwind, and so on. The good news is that you don’t need to learn all of them, though you probably should learn at least one of them. It’s up to you if you want to start by learning a framework before learning the vanilla technologies, but at some point you’ll want to be sure you’ve got some semblance of an understanding of the vanilla tech.
The vanilla tech means: HTML, CSS, and JavaScript. These are the basics that are part of 99.9% of every website. Many (arguably, most) websites can be built with some combination of a backend and a purely vanilla frontend. This is because of how far browser standards have evolved, enabling very powerful features out of the box.
HTML, CSS, and JavaScript are each very rich languages, but here are some examples of things you’ll want to learn as part of your journey:
Markup. Markup is the vocabulary you use to build a page. Things like div, span, p, h1, h2, section, article, nav, main, and so on. This mostly refers to tags, but also includes attributes on those tags.
HTML Structure. Each HTML page has (or can have) certain elements, such as the doctype, the head, the body, script tags, style tags, and other tags that offer more than simply visual building blocks.
Metadata. Beyond the data you can see on the page, there is data that is used for search engines (and other purposes). Examples include the page title, page description, canonical URLs, and structured data (e.g., company details like hours of operation).
Forms. Most websites have at least one form. While you can certainly have a purely visual website that displays data, it is very common to want a user to enter some data that is then processed in some way (e.g., a contact form that then sends an email).
Box Model / Borders / Outlines / Colors / Fonts. These are the basic styles you can apply to elements on the page.
Flexbox / Grid. CSS gives you a ton of options for laying out your content. Flexbox and grid are newer and very popular options.
Media Queries / Container Queries. These allow for your page to be adjusted based on other factors, such as screen size, screen type, and supported browser features.
CSS Custom Properties. These allow you to reuse and chain values rather than copying the same magic strings everywhere.
Variables. Variables in JavaScript are more complicated than in other languages due to how they evolved over time. You’ll want to understand var, let, and const, for a start.
Functions. Unlike most languages, JavaScript functions can act like classes (though JavaScript now supports classes outright). There are also other peculiar aspects to JavaScript functions, such as how they impact variable scoping and hoisting behaviors.
JSON. JSON is a subset of JavaScript that focuses on the structure of data objects.
DOM Operations. Browsers convert HTML into a more dynamic document that consists of a DOM. You can think of DOM as markup that can move. You can insert new items into the DOM, remove items, change the position, attach data, listen for changes, query for specific DOM elements, and so on.
JavaScript APIs. JavaScript has several built-in APIs, such as the history API, cryptography helpers, fetch, canvas, service workers, cookies, and so on.
Events. Much of JavaScript is driven by events. You can set timers, react to user input, and chain complex interactions to create hybrid events, as well as emit and respond to your own custom events.
Web Components. You can think of web components as being able to create your own markup with its own special behaviors and layout.
Accessibility. This is really worth its own section, but accessibility is becoming more crucial than ever to every developer who works on a website. In essence, accessibility refers to making websites more usable to those with disabilities. The most common accessibility standard now used is WCAG.
Page Speed. Something you may find when you build with frameworks is that you run into problems with a low page speed score. It takes a fairly deep understanding of multiple aspects of web development to ensure a good page speed score. Beyond just the score, a good page speed will help ensure happy users and good rankings on search engines.
There are many books on these, and they often combine all three topics into the same book, but occasionally a book will focus on just one (especially the case with JavaScript).
There are also entire books on very specialized topics, such as Three.js (a framework that renders 3D scenes to a canvas element). It might seem overwhelming when you consider that there are over 100 built-in HTML tags, and that there are entire books on topics relating to a single HTML tag. However, also consider that you don’t need to know everything (in this case, maybe you have no interest in 3D in the browser), and that most tags are quite simple and have very little to learn about them.
Choose a Frontend Framework
By no means do you need to be an expert in a frontend framework, but it is often the case that employers are looking for somebody skilled in the common frameworks. For business-oriented websites (lots of complexity and dynamic functionality), Angular is what people often choose (it includes a lot of functionality, but also a lot of bloat).
For many websites, React is chosen. React has the benefit that mastering it makes it easier to learn React Native, should you eventually want to build cross-platform mobile apps. React also has a robust ecosystem, so you can do quite a lot with it.
Where performance is a top priority, developers often go with Svelte or Solid. These are not the only frameworks out there, but they are very popular choices.
I don’t have a strong preference for any of these, but here is a video introduction to Solid since it’s one of the most succinct videos I’ve ever seen for these sorts of frameworks: https://www.youtube.com/watch?v=hw3Bx5vxKl0
When deciding between these frameworks, it can sometimes help to watch a quick video on each so you can get a gut feeling for what it would be like to work with them, and that can help you decide which you want to learn the most.
SQL Database Development
There are many ways to store and retrieve data. One of the simplest is to utilize the file system, but that is usually a part of learning most any backend language. For most serious websites, you’ll need something more robust than just plain files on the file system. This is where databases come into play.
While there are plenty of NoSQL databases available, you will most likely encounter SQL databases during your career, as they are used to some degree in a majority of companies. There are also many dialects of SQL, but when it comes to .NET you will mostly encounter SQL Server (which uses Transact-SQL). The good news is that most dialects of SQL share very similar syntaxes, so once you’ve learned one you’ve learned most of the others.
Another facet of working with databases and .NET is that you’ll probably want to utilize an ORM (object-relational mapper), and more specifically this ORM will more commonly be EF (entity framework). When working with EF, you will probably do so via LINQ, which is a special C# syntax that makes working with data easier (especially SQL data).
SQL allows you to store/insert data into the database, query for that data, update and delete the data, and a number of other things (such as modifying the structure of the data itself using SQL statements that can change tables, columns, views, and indexes).
ASP.NET MVC Backend
The main backend architecture used nowadays for projects utilizing C# is ASP.NET MVC. This really means you have a project with the following:
The language is C#.
The pattern is MVC, which stands for model view controller.
The view engine syntax is Razor.
I call Razor a syntax rather than a language because it is really a combination of several languages in one. It looks like HTML, you can incorporate C#, and there are special additions like tag helpers (basically, HTML with special server-side behaviors).
It is cross-platform, so you can build and host ASP.NET MVC websites using Linux, macOS, or Windows. When building on Windows, you will want to use Visual Studio or Rider. On other operating systems, I recommend Rider. Visual Studio Code is technically an option, but you will be missing out on a lot by not using one of the more robust IDEs.
There are plenty of books out there that will help you with ASP.NET MVC. Just be sure to get one that focuses on C# (there may still be some that use VB.NET).
Aim for a book that teaches best practices beyond just ASP.NET MVC itself. For example, it probably should cover n-tier architecture, which is where you split your code into multiple layers, such as a presentation layer, a business logic layer, and a data access layer.
A CMS as a Backend
Aside from social networks and functionality-heavy websites, most websites heavily feature content from one company, which is typically written by its employees. Since it would be wasteful to go to a developer each time they want to update some content (usually text, images, and videos), the most common solution is to utilize a CMS (content management system). This means that as a full stack developer, you will want to be sure you are comfortable with how to use a CMS.
A side effect of this is that you may often find it’s the case that you rely more on the CMS and the functionality it provides rather than working directly with ASP.NET MVC code. This is especially true now that headless CMSs are more popular nowadays. A headless CMS essentially provides APIs to access the content rather than serving webpages directly from the CMS website itself. This means that you’ll want to pick a CMS that offers a headless approach, just to be sure you’re familiar with how that works.
There are many options out there, but I’ll share some that I am familiar with. Umbraco would be my top choice. It is a free and open-source .NET CMS, and in more recent versions, it has included a headless option—several, in fact. One option is to use your own Umbraco install, which includes a somewhat limited headless API. The other is to use the paid cloud service called Umbraco Heartcore.
Another option you can consider is Contentful. This isn’t a .NET CMS, but because it is headless, the backend technology doesn’t really matter. Once you’ve learned how to interact with the (typically GraphQL) API of one headless CMS, that knowledge is readily transferrable to other headless CMSs.
If you are more interested in e-commerce, Shopify might be an option to consider. Shopify is another CMS that isn’t based on .NET (it uses more proprietary technologies), but it does have a headless option.
This is just scratching the surface, as there are hundreds of CMSs out there. The good news is that they often share many common features, so once you learn those they translate well to other CMSs. Here are some of those features:
Creating the structure of “documents” (e.g., all the fields related to a product).
Using a component-based approach to enable content editors to have flexible options when constructing pages.
Managing permissions.
Managing forms.
Managing media (images, videos, PDFs, and so on).
Indexing and searching content.
Once you do those things in a couple of different CMSs, you’ll start to see how they’re more alike than different. Some differences might catch you off guard, such as how one CMS may structure pages hierarchically and another may use a bucketed approach, but those are relatively trivial details once you understand the basics.
Node or Serverless Backend
One option some full stack developers choose is to use JavaScript as their backend in one way or another. This could be in the form of Express on a full server, a static site generator like Gatsby, or a framework like Next hosted with services like Netlify or Vercel.
The main advantage to this approach is that it doesn’t require you to learn another programming language (because you are using JavaScript on the backend). However, there are some disadvantages to consider:
In the case of static site generators, you may have trouble building more dynamic backend functionality, as they are more geared toward converting content into static HTML files. Implementing something like an authenticated member section could prove problematic.
In the case of Next, it may be hard to reason about what is backend vs what is frontend code, since they can sit side-by-side.
Many of these approaches assume a given frontend framework, such as React (very common) or Svelte. This means you may end up taking on the extra baggage of the framework (extra cognitive load, extra JavaScript bundle size) when it’s not actually needed for your specific use case.
Coupling the backend and frontend can sometimes seem initially to reduce complexity, when in fact it often introduces extra complexity, such as needing to hydrate a server side rendered frontend to ensure interactivity (though frameworks like Next do attempt to address this to minimize how much work you need to do to enable this).
If you accept these caveats, using JavaScript as your backend may be a valid approach to becoming a full stack developer.
A related architecture is called “serverless.” This is a peculiar name, as what it really means is “even more servers.” The way serverless tends to work is that, instead of having a single central server or a few load-balanced servers, many servers from around the world can be used.
Each platform is different, but these serverless servers generally use Node (server-side JavaScript), are stateless (i.e., you can’t persist data in-memory or to the file system that can be shared among multiple web requests), and can’t use much resources (e.g., they only offer limited RAM, limited CPU utilization, and will not allow long-running operations).
That said, they do offer a way to run JavaScript on the backend, even if the backend is distributed and limited. As a bonus, these serverless servers often run on the “edge” (meaning, in a server that is geographically near the website visitor, and so it will respond quicker to their requests), and the pricing models are generally favorable (since they don’t require underutilized hardware dedicated to a single customer).
If you are just starting out with all of this and want to get something up and running quickly, Next is a good option since it will handle some of the plumbing for you.
DevOps
Speaking of plumbing, that is essentially what DevOps is, at least within the context of being a full stack developer. While DevOps can mean a wide range of things, I’ll restrict my definition somewhat so that it is more relevant:
“DevOps is what you need to get your code in front of users.”
And in more concrete terms:
“The role of DevOps is to automatically deploy code to a web server from a Git repository.”
That leaves out a lot, but automated deployments are the most useful aspect to you as someone learning to be a full stack dev. If you are curious, some other aspects include: automated testing, provisioning (and deprovisioning) servers, securing infrastructure, managing backups, and monitoring infrastructure.
Many services and cloud providers attempt to streamline much of this so that as a developer you can focus mostly on building the code. That said, at some point or another, you’re going to have to learn one of these services/providers to at least get your code deployed somewhere. There are many options, but here are some of them:
TeamCity. This is a tool that allows you to run build and deploy tasks. There is a cloud option so you don’t need to worry about hosting it locally. This is just one among many CI/CD tools available on the market.
Netlify & Vercel. These services help more when your architecture is very JavaScript-heavy. They have built-in templates that remove some of the guesswork when configuring Next, Gatsby, serverless, and similar approaches.
GitHub Actions & Bitbucket Pipelines. These are akin to TeamCity, but come with their own limitations. If you are hosting in GitHub or Bitbucket, it may be worth trying these to see if they work for you.
GitHub Pages & Cloudflare Pages. If you want to start very simple, these are some services that will help you get a mostly static site up quickly and relatively painlessly. I sometimes use these for documentation websites or static sites that have a simple set of Node build steps. These could also be used for a portfolio website (as those are generally static).
No matter which tool you use, the end goal is that when you push a code change to a repository, the tool should automatically build and deploy that code to a web server.
Learning to use one or more of these tools will help you to get your websites in front of users, and to rapidly iterate so you can share your changes more frequently and with minimal effort on your part.
Suggested Next Steps
Now that you know what to learn, where do you start? Start with what interests you the most! If you lean more toward backend, perhaps start looking into C#. If you are into frontend, start learning the basics or jump into learning one of the frameworks. Then fill in the gaps as you go.
As mentioned, this could be in the form of subscribing to one of the learning websites, finding some YouTube videos, or reading some books. In the case of books, here are some options (I haven’t thoroughly vetted this list, so consider it to be inspiration for building your own list):
HTML, CSS, and JavaScript All in One: Covering HTML5, CSS3, and ES6, Sams Teach Yourself: https://www.amazon.com/HTML-JavaScript-Sams-Teach-Yourself/dp/0672338084/
Eloquent JavaScript, 3rd Edition: A Modern Introduction to Programming: https://www.amazon.com/Eloquent-JavaScript-3rd-Introduction-Programming/dp/1593279507/
Learn C# Programming: https://www.amazon.com/Learn-Programming-building-foundation-efficient/dp/1789805864/
SQL QuickStart Guide: https://www.amazon.com/SQL-QuickStart-Guide-Simplified-Manipulating/dp/1945051752/
ASP.NET Core in Action, Third Edition: https://www.amazon.com/ASP-NET-Core-Action-Third-Andrew/dp/1633438627/
Think Like a Programmer: An Introduction to Creative Problem Solving: https://www.amazon.com/Think-Like-Programmer-Introduction-Creative/dp/1593274246/
DevOps Unleashed with Git and GitHub: https://www.amazon.com/DevOps-Unleashed-Git-GitHub-collaborate/dp/1835463711/
React Key Concepts: https://www.amazon.com/React-Key-Concepts-Consolidate-knowledge/dp/1803234504/
A Common-Sense Guide to Data Structures and Algorithms, Second Edition: https://www.amazon.com/Common-Sense-Guide-Structures-Algorithms-Second/dp/1680507222/
Design Patterns in TypeScript: https://www.amazon.com/Design-Patterns-TypeScript-Implemented-Engineering/dp/B0948BCH24/
You can also look into some interactive roadmaps, such as this one (I don’t entirely agree with the items on here, but every journey will be different): https://roadmap.sh/full-stack
Another surprising place to refine your skills is on the leetcode.com website. You can choose the programming language you want to use and work through a vast library of small programming puzzles. As you go through them, you will learn more and more, even if you have already mastered a given programming language. While the problems you work on may not have much practical use (they often use obscure algorithms and problems that don’t relate well to the real world), they will help you to think like a programmer.